Code for your future selves, not your current self.
Code is read much more often than it is written, so plan accordingly
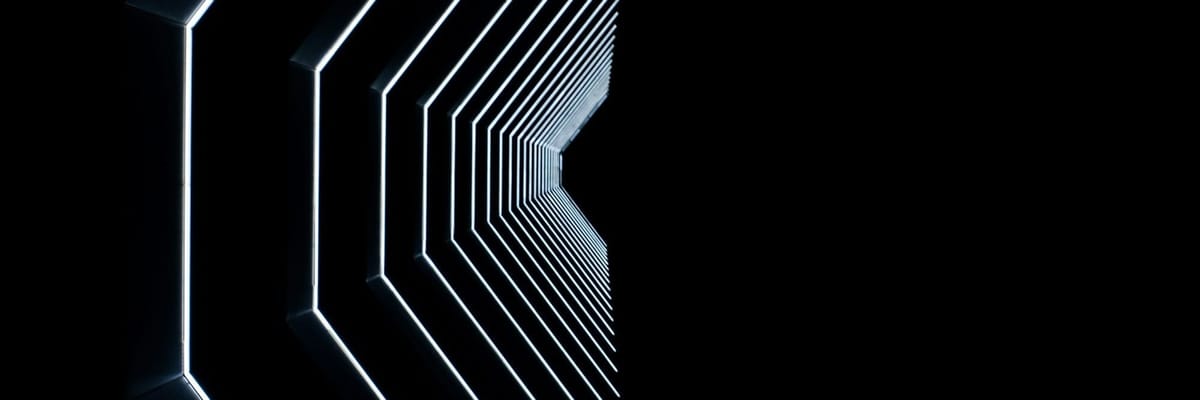
Code is read much more often than it is written, so plan accordingly
I recently had a conversation with an engineer on my team, when a fun thought came to mind.
The engineer had written some code that implemented an incredibly complex set of requirements. The module the engineer wrote was responsible for signing and cryptographically verifying communication between peers, in a distributed system, and so there was a fair amount of esotericism and complexity just in the cryptography, yet alone the implementation.
On top of this, the engineer had to implement this logic in a programming language he had only recently started to learn: Erlang. Erlang is a functional programming language that is designed to build highly available, fault tolerant, distributed systems, initially for the telecom space. Its design builds on top of the Actor Oriented programming paradigm, literally building the paradigm into the language semantics. In Erlang, components are modeled as independent "Actors" that orchestrate and communicate by sendings messages to each other. There is no shared state between Actors, all data is immutable, and all code flow is modeled using something called "Pattern Matching". For many developers, writing Erlang feels very strange, especially initially.
I also had to learn Erlang working on this project, and I actually really enjoyed it.
Code that I write tends to be more functional and compositional, which seems to have lent itself to my learning Erlang, but there is certainly a learning curve.
For example, there isn't even syntax for "for-loops". Instead, the way you'd model looping is with a recursive function.
For example, here's how you might implement a simple map
function, in Erlang:
map(_, []) -> []; % Return an empty list
map(F, [H | T]) -> % H matches the first elem (the head)
[F(H) | map(F, T)]. % Apply F to H, then recursively map the rest of the list T (the tail)
Luckily Erlang has tail-call optimization, something, whose lack of, i've had to work around in other programming languages (trampolines, anybody?)
Erlang is not a popular programming language – the problems it is really geared towards solving are problems most teams never have to deal with, and so there are not as many resources and a community around it compared to more popular programming languages like JavaScript, Python, or Java.
On top of all of this, the engineer was not a security expert. He was proficient, but his prior experience was mainly in game development.
So this engineer translated this incredibly complex set of requirements, in a problem domain that was not his expertise, into a program written in a weird language that he had only recently started to learn. If you're an engineer, you can appreciate the intensity and impressiveness of that last sentence – like, whoa. But it's about to get even more impressive.
Naturally, we had a few team members review his code. My story, and the start of my fun thought, has to do with what happened with the first team member.
Now the first team member to review the code was a security expert, an expert in cryptography with an extensive background building and auditing secure systems, and so would be able to spot any discrepancies in the cryptography of it all. But --this team member did not know Erlang, at all. How was he going to know what the code was even doing. Well luckily, the first engineer left a lot of comments.
The security engineer, by following the comments, was able to review the soundness of the cryptography and sign off on it. This team member told me about his experience:
"I've never done Erlang and was able to follow exactly the intention of this tricky flow – was very cool"
To me, this was truly amazing. Of course there were subsequent reviews on the soundness of the implementation itself, but this response simply blew me away. I had to reach out to the first engineer just to express thanks and a "Well done!". I spoke to him, and told him about what the security engineer expressed about his code – how it was easy to follow, because it was so well commented. His response was really interesting – he said something along the lines of:
"When I wrote those comments, they were mainly just for me, so that I could remember what the hell my code was even doing and why I wrote it the way I did."
And this is when my fun thought occurred.
If you're an engineer, you've definitely had the experience of revisiting code you wrote 1 month, 3 months, 1 year ago. You sit there, gawking at it, thinking: "what in the world was I thinking when I wrote this code?" Now that question could actually be asking many different things underneath the hood, but it often times is asking one of these two questions:
- "This code is awful. Why did I write such bad code?"
The answer there is simple: optimistically, you're a better engineer now. Pessimistically, you've become more dogmatic in your coding style. Either way, the solution is simple: refactor it, have a nice day.
The next question is more subtle, but the way more crucial one to ask:
- "Why did I write this code in this particular way? Why did I group these lines here and those over there? Why did separate these steps, and why did I choose this order? What meaning was I trying to convey? What in this code is significant?
The answer here is much more complex, and almost entirely depends on context. The requirements, the timeline, other team members, workarounds or technical debt that existed at the time – all of these factors and more will have influenced how you, an engineer, at that moment in time, decided to write that code. But where does all that context go after the code is written? The fact that you're asking the question proves that it is valuable information to have, at least with respect to understanding the code. Now, if only we had a code-native way of recording that context – hmm /s.
Software documentation, in the form of catalogs, READMEs, diagrams, even simple comments – they're always for posterity. Like, even the code itself is for posterity. The code is automating some thing so that a future something or someone doesn't have to manually do it. It is always for some future engineer.
My fun thought: that includes your future selves. After all, aren't you just an team of engineers, spread across time?
The best sort of code comments describe the WHY for a piece of code and potentially the WHAT, if it is not clear. Especially with external dependencies, since we don't have control over them, this is a potential hot spot for esoteric code, and deserves good comments. It is a good idea to leave comments describing 3rd party constraints, integrations, and quirks.
/**
* Describe the background
*
* Describe the problem
*
* Describe the decision/solution
*/
Here's a comment from a piece of code I recently wrote (In Erlang)
% In order to reproduce the body derived from the message,
% we need to generate a unique & reproducible
% multipart boundary for the multipart body.
%
% However we cannot use the id of the message as
% the boundary, as the id cannot be derived until
% the message is fully encoded.
%
% So we encode each part individually,
% concatenate them, SHA-256 hash, then base64url encode
% to produce our multipart boundary.
%
% This ensures that the boundary is unique, reproducible, and
% secure.
Here's another comment from a piece of code I wrote 2 years ago (In JavaScript)
/**
* When using with AWS s3 and coupled with the fact
* that LocationConstraint is not required _only_ for us-east-1
(https://docs.aws.amazon.com/AmazonS3/latest/API/API_CreateBucketConfiguration.html),
* this can cause some hair-pulling gotchas when creating a bucket
* and not passing region -- the bucket will automatically
* be attempted to be created in us-east-1, REGARDLESS of whichever
* region used to instantiate the MinIO Client.
*
* So if you're using something like IAM to securely access s3, or a VPC
* Endpoint for s3 in a region that is _not_ us-east-1,
* you will simply get an opaque S3 AccessDenied error when creating the
* bucket -- your IAM Role might be constrained to only
* access, say us-east-2, or your VPC Endpoint is for
* s3.us-east-2.amazonaws.com, and accessing us-east-1 out of the blue
* will simply produce a seemingly random "AccessDenied". -_______-
*
* SO, we MUST pass region here that is provided to the adapter,
* to ensure the bucket is created in the desired region,
* and any credentials imbued by IAM or the VPC are used.
*/
With that comment, my future self can understand why my past self made the decisions and wrote the code that way. Without this comment, I may be doomed to repeat the same mistake, or not realize what needs to be there, and what can be removed/refactored – what is significant.
Comments are not for you, now. They're for your fellow team of engineers, later. That includes future "yous", the team of engineers, across time. Do yourselves a favor and comment your freaking code.